Download+Install Basic Usage Digital I/O PWM & Tone Timing USB Serial USB Keyboard USB Mouse USB Joystick USB MIDI. OneWire Library. Dallas Temperature Control Library. Arduino's OneWire page (warning: has buggy version). ESP8266 core for Arduino. Contribute to esp8266/Arduino development by creating an account on GitHub. Arduino / libraries / Wire / Wire.h. 22bab5f Oct 28, 2018. Devyte Fix arg type in Wire to size_t. This library is distributed in the hope that it will be useful. Once you download the library ZIP file you’ll need to install it in your Arduino IDE. To do this follow these instructions: Open the Arduino IDE. Click on the Sketch menu at the top of the IDE. Choose Include Library from the drop-down menu. Select Add.ZIP Library from the sub-menu. A dialog box will open.
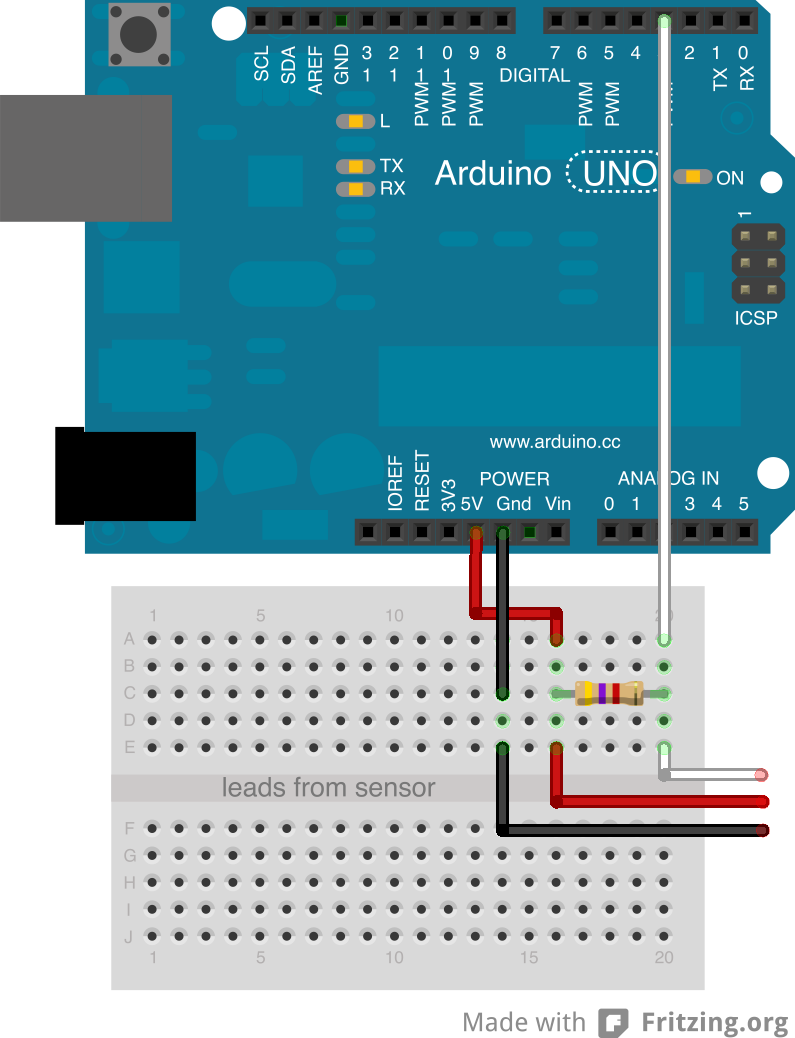
This add-on is supported for MATLAB R2016a - R2018b.
This add-on extends MATLAB Support Package for Arduino Hardware to read from and write to 1-Wire devices. With the add-on, you can reset the device, read or write a single byte or multiple bytes from or to the device and also check the data integrity using either CRC8 or CRC16 algorithm.
Sample usage:
-------------------
% Create arduino object with the add-on library
a = arduino('COM38', 'Uno', 'Libraries', 'PaulStoffregen/OneWire');
% Create 1-Wire object
sensor = addon(a, 'PaulStoffregen/OneWire');
% Obtain sensor ROM address
addr = sensor.AvailableAddresses{1};
% Reset sensor
reset(sensor);
% Write to sensor
write(sensor, addr, 72, 1) % parasite power on
% Read 9 bytes from sensor
read(sensor, addr, 9)
% Check CRC of received data
checkCRC(sensor, [1 2 3 4], 56, ‘crc8’)
It includes documentation and an example that demonstrates the use with a DS18B20 temperature sensor and DS2431 1024-bit EEPROM.
Important: Before using this add-on library in MATLAB, you need to install the OneWire Arduino library. Here are the instructions:
1. Download the zip file from https://github.com/PaulStoffregen/OneWire/archive/v2.3.2.zip
2. Unpackage the zip into local directory and rename the folder to ‘OneWire’.
3. Move the ‘OneWire’ folder into the 'libraries' folder inside your Arduino sketchbook folder:
On Windows, the default path is 'My DocumentsArduinolibraries'
On Mac, the default path is '~/Documents/Arduino/libraries/'
On Linux, the default path is '/home/<username>/Arduino/libraries'
Troubleshooting tips:
1. After installing this add-on library and the required Arduino library, type 'listArduinoLibraries' in MATLAB to see if the add-on is properly installed.
2. Wire up the Arduino board and the add-on device properly before creating the object to avoid a connection error in MATLAB.
3. Refer to the documentation for details on usage and syntax. It can be found under Supplemental Software in the product documentation.
Feel free to contact the MATLAB Hardware Team if you have questions about this add-on library:
http://www.mathworks.com/matlabcentral/profile/contact/4922363-mathworks-matlab-hardware-team
Join GitHub today
GitHub is home to over 40 million developers working together to host and review code, manage projects, and build software together.
Sign up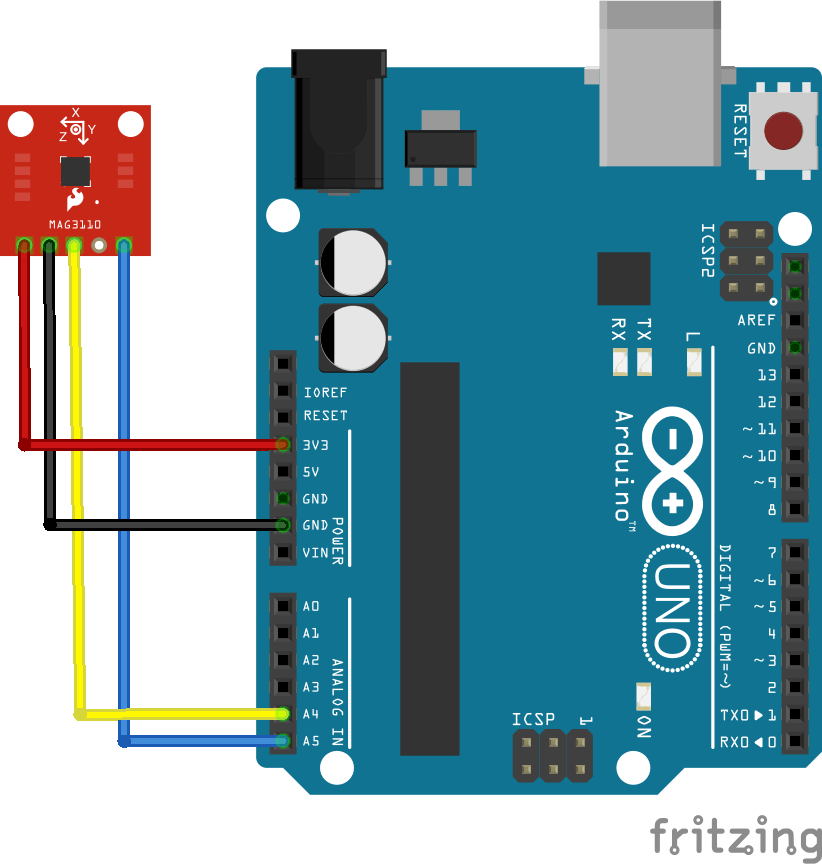
8 contributors
Arduino Code Library
/* |
TwoWire.h - TWI/I2C library for Arduino & Wiring |
Copyright (c) 2006 Nicholas Zambetti. All right reserved. |
This library is free software; you can redistribute it and/or |
modify it under the terms of the GNU Lesser General Public |
License as published by the Free Software Foundation; either |
version 2.1 of the License, or (at your option) any later version. |
This library is distributed in the hope that it will be useful, |
but WITHOUT ANY WARRANTY; without even the implied warranty of |
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU |
Lesser General Public License for more details. |
You should have received a copy of the GNU Lesser General Public |
License along with this library; if not, write to the Free Software |
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA |
Modified 2012 by Todd Krein (todd@krein.org) to implement repeated starts |
Modified December 2014 by Ivan Grokhotkov (ivan@esp8266.com) - esp8266 support |
Modified April 2015 by Hrsto Gochkov (ficeto@ficeto.com) - alternative esp8266 support |
*/ |
#ifndef TwoWire_h |
#defineTwoWire_h |
#include<inttypes.h> |
#include'Stream.h' |
#defineBUFFER_LENGTH128 |
classTwoWire : publicStream |
{ |
private: |
staticuint8_t rxBuffer[]; |
staticuint8_t rxBufferIndex; |
staticuint8_t rxBufferLength; |
staticuint8_t txAddress; |
staticuint8_t txBuffer[]; |
staticuint8_t txBufferIndex; |
staticuint8_t txBufferLength; |
staticuint8_t transmitting; |
staticvoid (*user_onRequest)(void); |
staticvoid (*user_onReceive)(size_t); |
staticvoidonRequestService(void); |
staticvoidonReceiveService(uint8_t*, size_t); |
public: |
TwoWire(); |
voidbegin(int sda, int scl); |
voidbegin(int sda, int scl, uint8_t address); |
voidpins(int sda, int scl) __attribute__((deprecated)); // use begin(sda, scl) in new code |
voidbegin(); |
voidbegin(uint8_t); |
voidbegin(int); |
voidsetClock(uint32_t); |
voidsetClockStretchLimit(uint32_t); |
voidbeginTransmission(uint8_t); |
voidbeginTransmission(int); |
uint8_tendTransmission(void); |
uint8_tendTransmission(uint8_t); |
size_trequestFrom(uint8_t address, size_t size, bool sendStop); |
uint8_tstatus(); |
uint8_trequestFrom(uint8_t, uint8_t); |
uint8_trequestFrom(uint8_t, uint8_t, uint8_t); |
uint8_trequestFrom(int, int); |
uint8_trequestFrom(int, int, int); |
virtualsize_twrite(uint8_t); |
virtualsize_twrite(constuint8_t *, size_t); |
virtualintavailable(void); |
virtualintread(void); |
virtualintpeek(void); |
virtualvoidflush(void); |
voidonReceive( void (*)(int) ); // arduino api |
voidonReceive( void (*)(size_t) ); // legacy esp8266 backward compatibility |
voidonRequest( void (*)(void) ); |
using Print::write; |
}; |
#if !defined(NO_GLOBAL_INSTANCES) && !defined(NO_GLOBAL_TWOWIRE) |
extern TwoWire Wire; |
#endif |
#endif |
Arduino Projects
Copy lines Copy permalink